error.js
错误文件允许你处理意外的运行时错误并显示回退 UI。
¥An error file allows you to handle unexpected runtime errors and display fallback UI.
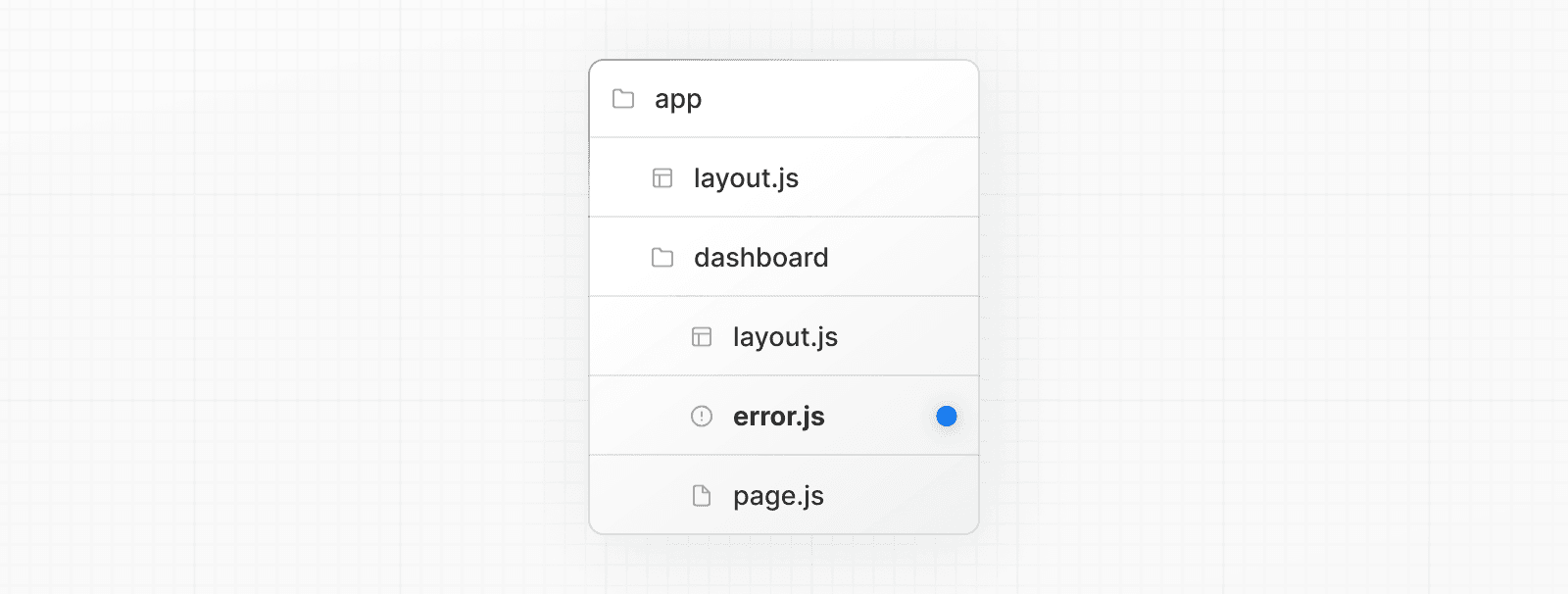
'use client' // Error boundaries must be Client Components
import { useEffect } from 'react'
export default function Error({
error,
reset,
}: {
error: Error & { digest?: string }
reset: () => void
}) {
useEffect(() => {
// Log the error to an error reporting service
console.error(error)
}, [error])
return (
<div>
<h2>Something went wrong!</h2>
<button
onClick={
// Attempt to recover by trying to re-render the segment
() => reset()
}
>
Try again
</button>
</div>
)
}
error.js
将路由段及其嵌套子项封装在 React 误差边界 中。当边界内出现错误时,error
组件将显示为后备 UI。
¥error.js
wraps a route segment and its nested children in a React Error Boundary. When an error throws within the boundary, the error
component shows as the fallback UI.
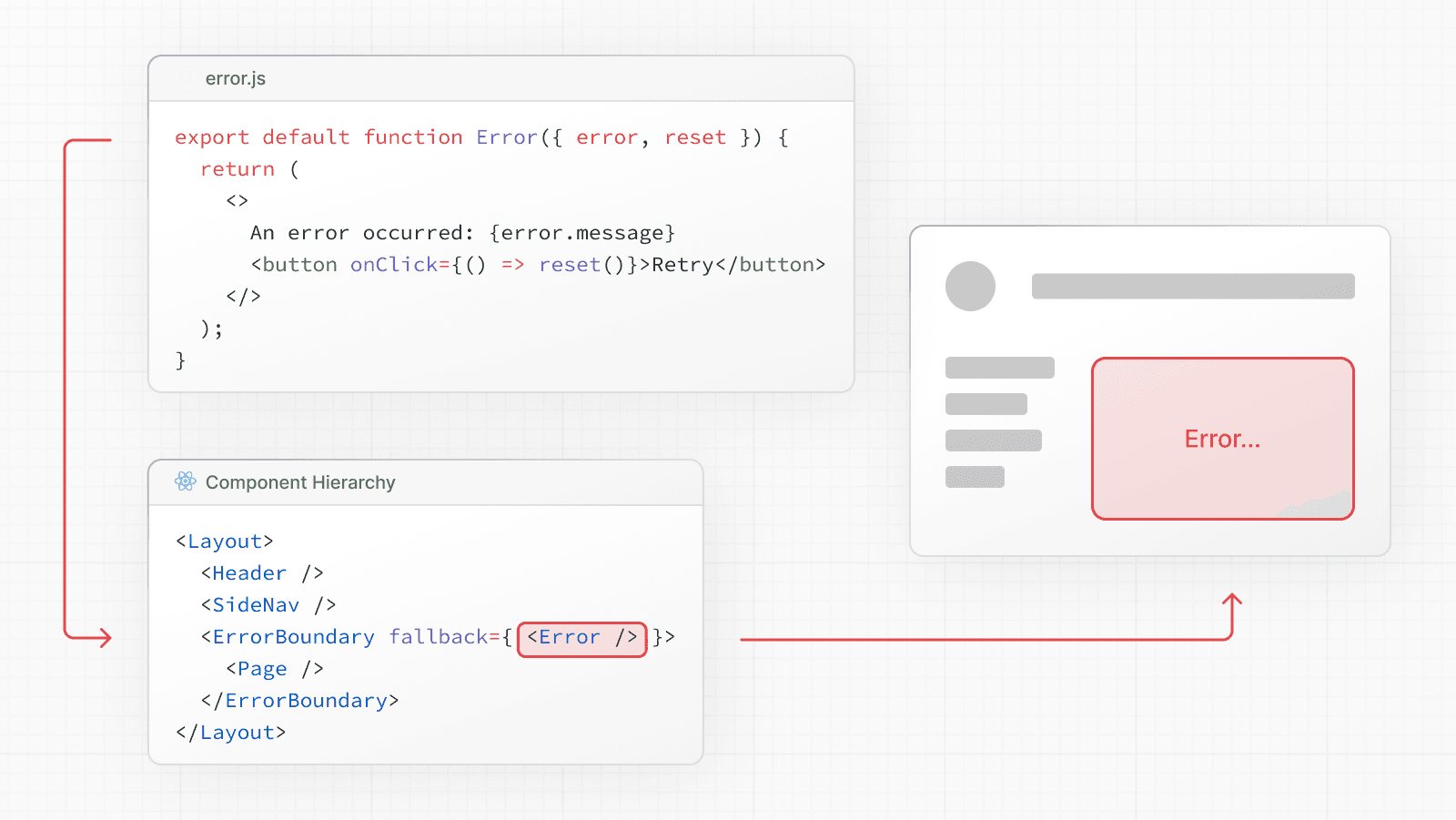
需要了解:
¥Good to know:
React DevTools 允许你切换错误边界以测试错误状态。
¥The React DevTools allow you to toggle error boundaries to test error states.
如果你希望错误冒泡到父错误边界,你可以在渲染
error
组件时使用throw
。¥If you want errors to bubble up to the parent error boundary, you can
throw
when rendering theerror
component.
参考
¥Reference
属性
¥Props
error
转发到 error.js
客户端组件的 Error
对象的实例。
¥An instance of an Error
object forwarded to the error.js
Client Component.
需要了解:在开发过程中,转发到客户端的
Error
对象将被序列化,并包含原始错误的message
,以便于调试。但是,这种行为在生产中有所不同,以避免将错误中包含的潜在敏感细节泄露给客户端。¥Good to know: During development, the
Error
object forwarded to the client will be serialized and include themessage
of the original error for easier debugging. However, this behavior is different in production to avoid leaking potentially sensitive details included in the error to the client.
error.message
从客户端组件转发的错误显示原始
Error
消息。¥Errors forwarded from Client Components show the original
Error
message.从服务器组件转发的错误显示带有标识符的通用消息。这是为了防止泄露敏感细节。你可以在
errors.digest
下使用标识符来匹配相应的服务器端日志。¥Errors forwarded from Server Components show a generic message with an identifier. This is to prevent leaking sensitive details. You can use the identifier, under
errors.digest
, to match the corresponding server-side logs.
error.digest
自动生成的抛出错误的哈希值。可以用来匹配服务器端日志中相应的错误。
¥An automatically generated hash of the error thrown. It can be used to match the corresponding error in server-side logs.
reset
错误的原因有时可能是暂时的。在这些情况下,重试可能会解决问题。
¥The cause of an error can sometimes be temporary. In these cases, trying again might resolve the issue.
错误组件可以使用 reset()
函数来提示用户尝试从错误中恢复。执行时,该函数将尝试重新渲染错误边界的内容。如果成功,后备错误组件将替换为重新渲染的结果。
¥An error component can use the reset()
function to prompt the user to attempt to recover from the error. When executed, the function will try to re-render the error boundary's contents. If successful, the fallback error component is replaced with the result of the re-render.
'use client' // Error boundaries must be Client Components
export default function Error({
error,
reset,
}: {
error: Error & { digest?: string }
reset: () => void
}) {
return (
<div>
<h2>Something went wrong!</h2>
<button onClick={() => reset()}>Try again</button>
</div>
)
}
示例
¥Examples
全局错误
¥Global Error
虽然不太常见,但你可以使用位于根应用目录中的 global-error.js
来处理根布局或模板中的错误,即使利用 internationalization 也是如此。全局错误 UI 必须定义自己的 <html>
和 <body>
标签。此文件在活动时替换根布局或模板。
¥While less common, you can handle errors in the root layout or template using global-error.js
, located in the root app directory, even when leveraging internationalization. Global error UI must define its own <html>
and <body>
tags. This file replaces the root layout or template when active.
'use client' // Error boundaries must be Client Components
export default function GlobalError({
error,
reset,
}: {
error: Error & { digest?: string }
reset: () => void
}) {
return (
// global-error must include html and body tags
<html>
<body>
<h2>Something went wrong!</h2>
<button onClick={() => reset()}>Try again</button>
</body>
</html>
)
}
使用自定义错误边界进行优雅的错误恢复
¥Graceful error recovery with a custom error boundary
当客户端渲染失败时,显示上次已知的服务器渲染的 UI 以获得更好的用户体验会很有用。
¥When rendering fails on the client, it can be useful to show the last known server rendered UI for a better user experience.
GracefullyDegradingErrorBoundary
是一个自定义错误边界的示例,它可以在发生错误之前捕获并保存当前 HTML。如果发生渲染错误,它会重新渲染捕获的 HTML 并显示持久通知栏以通知用户。
¥The GracefullyDegradingErrorBoundary
is an example of a custom error boundary that captures and preserves the current HTML before an error occurs. If a rendering error happens, it re-renders the captured HTML and displays a persistent notification bar to inform the user.
'use client'
import React, { Component, ErrorInfo, ReactNode } from 'react'
interface ErrorBoundaryProps {
children: ReactNode
onError?: (error: Error, errorInfo: ErrorInfo) => void
}
interface ErrorBoundaryState {
hasError: boolean
}
export class GracefullyDegradingErrorBoundary extends Component<
ErrorBoundaryProps,
ErrorBoundaryState
> {
private contentRef: React.RefObject<HTMLDivElement>
constructor(props: ErrorBoundaryProps) {
super(props)
this.state = { hasError: false }
this.contentRef = React.createRef()
}
static getDerivedStateFromError(_: Error): ErrorBoundaryState {
return { hasError: true }
}
componentDidCatch(error: Error, errorInfo: ErrorInfo) {
if (this.props.onError) {
this.props.onError(error, errorInfo)
}
}
render() {
if (this.state.hasError) {
// Render the current HTML content without hydration
return (
<>
<div
ref={this.contentRef}
suppressHydrationWarning
dangerouslySetInnerHTML={{
__html: this.contentRef.current?.innerHTML || '',
}}
/>
<div className="fixed bottom-0 left-0 right-0 bg-red-600 text-white py-4 px-6 text-center">
<p className="font-semibold">
An error occurred during page rendering
</p>
</div>
</>
)
}
return <div ref={this.contentRef}>{this.props.children}</div>
}
}
export default GracefullyDegradingErrorBoundary
版本历史
¥Version History
版本 | 更改 |
---|---|
v15.2.0 | global-error 也在开发中。 |
v13.1.0 | global-error 已引入。 |
v13.0.0 | error 已引入。 |