安装
¥Installation
系统要求
¥System requirements
开始之前,请确保你的系统满足以下要求:
¥Before you begin, make sure your system meets the following requirements:
Node.js 18.18 或更高版本。
¥Node.js 18.18 or later.
macOS、Windows(包括 WSL)或 Linux。
¥macOS, Windows (including WSL), or Linux.
自动安装
¥Automatic installation
创建新 Next.js 应用的最快方法是使用 create-next-app
,它会为你自动设置一切。要创建项目,请运行:
¥The quickest way to create a new Next.js app is using create-next-app
, which sets up everything automatically for you. To create a project, run:
npx create-next-app@latest
安装时,你将看到以下提示:
¥On installation, you'll see the following prompts:
What is your project named? my-app
Would you like to use TypeScript? No / Yes
Would you like to use ESLint? No / Yes
Would you like to use Tailwind CSS? No / Yes
Would you like your code inside a `src/` directory? No / Yes
Would you like to use App Router? (recommended) No / Yes
Would you like to use Turbopack for `next dev`? No / Yes
Would you like to customize the import alias (`@/*` by default)? No / Yes
What import alias would you like configured? @/*
出现提示后,create-next-app
将以你的项目名称创建一个文件夹并安装所需的依赖。
¥After the prompts, create-next-app
will create a folder with your project name and install the required dependencies.
手动安装
¥Manual installation
要手动创建新的 Next.js 应用,请安装所需的包:
¥To manually create a new Next.js app, install the required packages:
npm install next@latest react@latest react-dom@latest
然后,将以下脚本添加到你的 package.json
文件:
¥Then, add the following scripts to your package.json
file:
这些脚本涉及开发应用的不同阶段:
¥These scripts refer to the different stages of developing an application:
next dev
:启动开发服务器。¥
next dev
: Starts the development server.next build
:构建用于生产环境的应用。¥
next build
: Builds the application for production.next start
:启动生产服务器。¥
next start
: Starts the production server.next lint
:运行 ESLint。¥
next lint
: Runs ESLint.
创建 app
目录
¥Create the app
directory
Next.js 使用文件系统路由,这意味着应用中的路由由你构建文件的方式决定。
¥Next.js uses file-system routing, which means the routes in your application are determined by how you structure your files.
创建 app
文件夹。然后,在 app
内部创建一个 layout.tsx
文件。此文件为 根布局。它是必需的,并且必须包含 <html>
和 <body>
标签。
¥Create an app
folder. Then, inside app
, create a layout.tsx
file. This file is the root layout. It's required and must contain the <html>
and <body>
tags.
export default function RootLayout({
children,
}: {
children: React.ReactNode
}) {
return (
<html lang="en">
<body>{children}</body>
</html>
)
}
创建一个包含一些初始内容的主页 app/page.tsx
:
¥Create a home page app/page.tsx
with some initial content:
export default function Page() {
return <h1>Hello, Next.js!</h1>
}
当用户访问应用的根目录 (/
) 时,layout.tsx
和 page.tsx
都会被渲染。
¥Both layout.tsx
and page.tsx
will be rendered when the user visits the root of your application (/
).
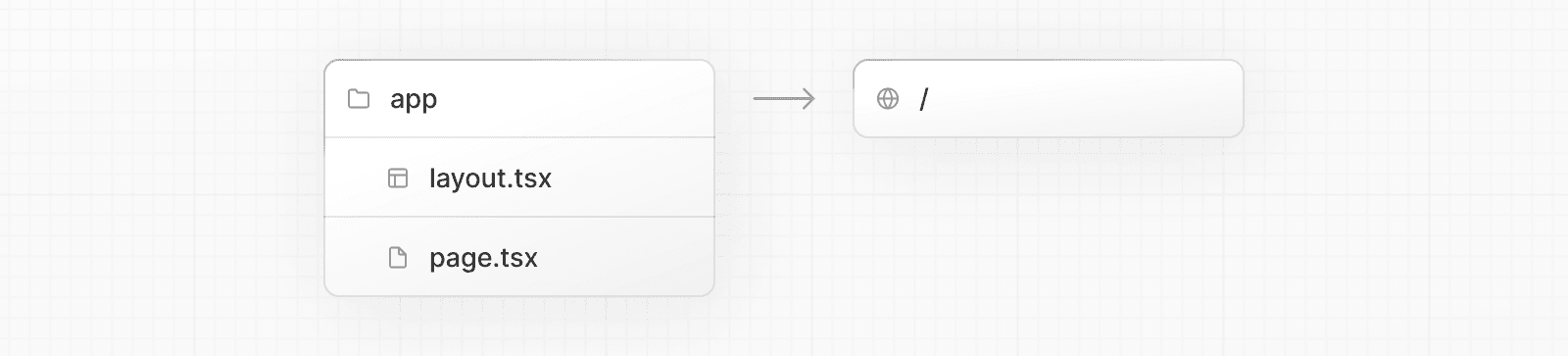
需要了解:
¥Good to know:
如果你忘记创建根布局,Next.js 将在使用
next dev
运行开发服务器时自动创建此文件。¥If you forget to create the root layout, Next.js will automatically create this file when running the development server with
next dev
.你可以选择在项目的根目录中使用
src
文件夹 将应用的代码与配置文件分开。¥You can optionally use a
src
folder in the root of your project to separate your application's code from configuration files.
创建 public
文件夹(可选)
¥Create the public
folder (optional)
在项目根目录创建一个 public
文件夹,用于存储静态资源,例如图片、字体等。然后,你的代码可以从基本 URL(/
)开始引用 public
中的文件。
¥Create a public
folder at the root of your project to store static assets such as images, fonts, etc. Files inside public
can then be referenced by your code starting from the base URL (/
).
然后,你可以使用根路径 (/
) 引用这些资源。例如,public/profile.png
可以引用为 /profile.png
:
¥You can then reference these assets using the root path (/
). For example, public/profile.png
can be referenced as /profile.png
:
import Image from 'next/image'
export default function Page() {
return <img src="https://nextjs.org/_next/image?url=https://h8DxKfmAPhn8O0p3.public.blob.vercel-storage.com/docs/light/typescript-command-palette.png&w=3840&q=75"/>
请参阅 [TypeScript 参考](/docs/app/api-reference/config/next-config-js/typescript) 页了解更多信息。
¥See the [TypeScript reference](/docs/app/api-reference/config/next-config-js/typescript) page for more information.
## 设置 ESLint {#set-up-eslint}
¥Set up ESLint
Next.js 内置 ESLint。当你使用 `create-next-app` 创建新项目时,它会自动安装必要的包并配置正确的设置。
¥Next.js comes with built-in ESLint. It automatically installs the necessary packages and configures the proper settings when you create a new project with `create-next-app`.
要手动将 ESLint 添加到现有项目,请将 `next lint` 作为脚本添加到 `package.json`:
¥To manually add ESLint to an existing project, add `next lint` as a script to `package.json`:
然后,运行 `npm run lint`,你将被引导完成安装和配置过程。
¥Then, run `npm run lint` and you will be guided through the installation and configuration process.
```bash filename="Terminal"
npm run lint
你会看到这样的提示:
¥You'll see a prompt like this:
? 你希望如何配置 ESLint?
¥? How would you like to configure ESLint?
❯ 严格(推荐)基础取消
¥❯ Strict (recommended)\ Base\ Cancel
严格:包括 Next.js 的基本 ESLint 配置以及更严格的 Core Web Vitals 规则集。这是首次设置 ESLint 的开发者的推荐配置。
¥Strict: Includes Next.js' base ESLint configuration along with a stricter Core Web Vitals rule-set. This is the recommended configuration for developers setting up ESLint for the first time.
基础:包括 Next.js 的基本 ESLint 配置。
¥Base: Includes Next.js' base ESLint configuration.
取消:跳过配置。如果你计划设置自己的自定义 ESLint 配置,请选择此选项。
¥Cancel: Skip configuration. Select this option if you plan on setting up your own custom ESLint configuration.
如果选择了 Strict
或 Base
,Next.js 会自动将 eslint
和 eslint-config-next
安装为应用中的依赖,并在项目根目录中创建包含所选配置的 .eslintrc.json
文件。
¥If Strict
or Base
are selected, Next.js will automatically install eslint
and eslint-config-next
as dependencies in your application and create an .eslintrc.json
file in the root of your project that includes your selected configuration.
现在,你每次想要运行 ESLint 来捕获错误时都可以运行 next lint
。一旦 ESLint 设置完毕,它也会在每次构建 (next build
) 期间自动运行。错误将使构建失败,而警告则不会。
¥You can now run next lint
every time you want to run ESLint to catch errors. Once ESLint has been set up, it will also automatically run during every build (next build
). Errors will fail the build, while warnings will not.
请参阅 ESLint 插件 页了解更多信息。
¥See the ESLint Plugin page for more information.
设置绝对导入和模块路径别名
¥Set up Absolute Imports and Module Path Aliases
Next.js 内置支持 tsconfig.json
和 jsconfig.json
文件的 "paths"
和 "baseUrl"
选项。
¥Next.js has in-built support for the "paths"
and "baseUrl"
options of tsconfig.json
and jsconfig.json
files.
这些选项允许你将项目目录别名为绝对路径,从而使导入模块更加轻松简洁。例如:
¥These options allow you to alias project directories to absolute paths, making it easier and cleaner to import modules. For example:
要配置绝对导入,请将 baseUrl
配置选项添加到你的 tsconfig.json
或 jsconfig.json
文件中。例如:
¥To configure absolute imports, add the baseUrl
configuration option to your tsconfig.json
or jsconfig.json
file. For example:
除了配置 baseUrl
路径之外,你还可以使用 "paths"
选项来配置 "alias"
模块路径。
¥In addition to configuring the baseUrl
path, you can use the "paths"
option to "alias"
module paths.
例如,以下配置将 @/components/*
映射到 components/*
:
¥For example, the following configuration maps @/components/*
to components/*
:
每个 "paths"
都相对于 baseUrl
位置。
¥Each of the "paths"
are relative to the baseUrl
location.